Introduction
This week, I want to share some of my knowledge on how to retrieve data from the Splinterlands API and Hive blockchain. I'll walk you through a small Python program that demonstrates how to use these APIs to analyze your lost battles.
This guide is meant to show what’s possible with Python and publicly available APIs, and while it’s just for fun, it opens the door to some interesting insights about your battles in Splinterlands.
Some of the biggest bot services operate using tokens (XBOT and ARCHMAGE). By analyzing your lost battles, you can check if your opponents have one or more of these tokens in their wallet. This doesn’t guarantee they are using a bot, but it might give you a clue.
I noticed my account had lost nine battles in a row, and I started to wonder: am I losing to better players, bots, or something else? This tool helps answer that question, but keep in mind that a player might hold bot tokens and still play the game manually.
Additionally, there are other bot services not covered here.
Setup python and retrieve SPL token
To get started, make sure you have Python installed. Then, you can install the necessary packages with this command:
pip install pandas requests hiveengine retrying
Next, you'll need to retrieve your own Splinterlands token. Follow these steps:
- Open the Splinterlands website Right-click anywhere on the page and select Inspect to open the developer tools.
- Go to the Network tab in the developer tools, and filter the results for the token.
⚠️ Important: Never share your token with anyone!
Once you have your token, update the Python script with your parameters:
account = 'ACCOUNT_NAME_TO_INSPECT_LOST_BATTLES'
token_params = {'token': 'YOUR_SPL_TOKEN', 'username': 'YOUR_SPL_USERNAME'}
Complete Script
Here's the complete Python script that analyzes your lost battles and inspects your opponents wallets:
import pandas as pd
import requests
from hiveengine.api import Api
from hiveengine.wallet import Wallet
from retrying import retry
account = 'ACCOUNT_NAME_TO_INSPECT_LOST_BATTLES'
token_params = {'token': 'YOUR_SPL_TOKEN', 'username': 'YOUR_SPL_USERNAME'}
PRIMARY_URL = 'https://api2.hive-engine.com/rpc/'
base_url = 'https://api2.splinterlands.com/'
def get_battle_history_df(account_name):
address = base_url + 'battle/history2'
params = token_params
params['player'] = account_name
params['limit'] = 50
wild_df = pd.DataFrame()
wild_result = requests.get(address, params=params)
if wild_result.status_code == 200:
wild_df = pd.DataFrame(wild_result.json()['battles'])
if wild_df.empty:
return None
else:
return wild_df
@retry(wait_exponential_multiplier=1000, wait_exponential_max=10000, stop_max_attempt_number=5)
def get_wallet_list(lost_battle_user, api):
# This function will retry up to 5 times with an increasing delay
try:
wallet_list = pd.DataFrame(Wallet(lost_battle_user, api=api)).sort_values(by=['symbol'])
return wallet_list
except Exception as e:
print(f"Service unavailable, retrying... ({str(e)})")
raise # This will trigger the retry
def main():
battles = get_battle_history_df(account)
# Determine which battles are lost (DRAWS will also be removed)
lost_battles = battles.loc[(battles.winner != account) & (battles.winner != 'DRAW')]
# Make list of all opponent you lost against
opponents = lost_battles.winner.tolist()
api = Api(url=PRIMARY_URL)
xbot = 0
archmage = 0
possible_human_or_other_bot = 0
undetermined = 0
for opponent in opponents:
wallet_list = pd.DataFrame()
try:
# Get wallet of opponent
wallet_list = get_wallet_list(opponent, api)
except Exception as e:
print(f"Failed to retrieve data after multiple attempts: {e}")
# Filter out XBOT tokens might be XBOT/XBOTLITE/XBOTMINI
xbot_df = wallet_list[
(wallet_list['symbol'].str.startswith('XBOT')) & (wallet_list['stake'].astype(float) >= 1)]
# Filter out ARCMAGE tokens might be ARCHEMAGE/ARCHEMAGEA/ARCHMAGEB
archmage_df = wallet_list[
(wallet_list['symbol'].str.startswith('ARCHMAGE')) & (wallet_list['balance'].astype(float) >= 1)]
# If the account has both tokens undetermined which bot service is used
if not xbot_df.empty and not archmage_df.empty:
undetermined += 1
print('Both tokens found not sure which is active.')
# If only xbot assume it has used the xbot service
elif not xbot_df.empty:
xbot += 1
# If only archmage assume it has used the xbot service
elif not archmage_df.empty:
archmage += 1
# Else it might be another bot service or human player
else:
possible_human_or_other_bot += 1
print(f'XBOT: {str(xbot)}')
print(f'ARCHMAGE: {str(archmage)}')
print(f'ARCHMAGE OR XBOT: {str(undetermined)}')
print(f'Maybe human or other bot: {str(possible_human_or_other_bot)}')
main()
Results
Over the past few days, I’ve been running this script and collecting data. My plan is to run the bot every other day analyzing 50 battles (which is the limit of the api) and my account plays 24 battles per day.
Here are some results:
Lost against bot service | Day 1 | Day 3 | Day 5 | Total |
---|---|---|---|---|
XBOT | 11 | 14 | 16 | 41 |
ARCHMAGE | 9 | 6 | 7 | 22 |
Both (undetermined) | 1 | 3 | 2 | 6 |
Maybe human or other bot | 7 | 0 | 0 | 7 |
Analyzing Lost Battles
This example highlights just one way you can use the Splinterlands and Hive APIs to analyze your battles. You can also dive deeper into specific details, such as identifying which teams consistently lose against certain cards or strategies.
I've developed a free, open-source tool that performs some of these deeper analyses and more. If you're interested in exploring these features and learning how to take your game analysis to the next level, feel free to check out my detailed post here:
Free Open-Source Splinterlands Statistics Tool
Closing notes
The intention of this post is purely educational, showing how easy it can be to use public APIs for analysis. I hope you found this content informative and fun!
If you enjoyed this post and would like to see more content like this, feel free to let me know. Also, if you have any ideas for tools or features you’d like me to explore or build, drop me a comment—I’d love to give it a try!
That's all for this week hope you enjoyed reading this post. See you all on the battlefield
Do you also want to be part of this amazing play to earn game consider using my refferal link.
Delegate Tokens and HP to Fallen Angels to earn weekly rewards!
Delegate | Join to the guildThis post has been supported by @fallen.angels guild!
Cool stuff. I generally just assume Wild is all bots, but I keep hearing rumors about madmen who play there manually :)
Nice to see examples of hiveengine calls. That is something I have been thinking about exploring but have not gotten around to yet.
Thanks @kalkulus, yeah fun stuff to explore :)
Yes, lots of bots in Wild now - which is also interesting because so many bots make it unlikely to have new winner teams created (bots feed on existing data, they can't create teams on their own), but that doesn't really happen :-D The new reward cards for example are taking a while to be included, even though they're some really amazing ones among them.
Thanks for the post!
Thanks @beelzael,
Agree, bots are using teams based its own data. Only when you lose against a new meta team the bot will learn and adapt i think.
Thanks for sharing! - @clove71
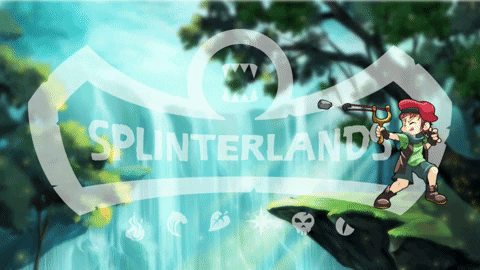
Thanks @clove71