To start, I have no idea where my Owner/Master Key for my @wlffreitas account is, so I'm posting through this old account.
Today I want to share a simple idea that could be useful for those developing in Godot and want to integrate Hive Blockchain data into their project. I'll show a step-by-step process for retrieving information from a Hive account, all using the public API from Hive Explorer and Godot.
https://hivexplorer.com/api-docs
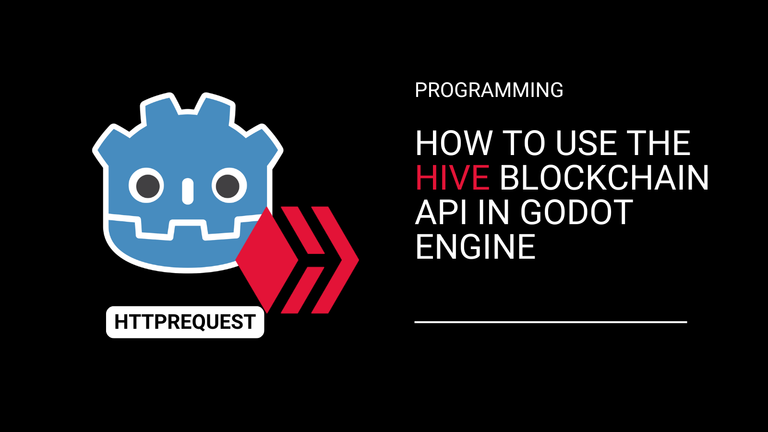
I won't waste too much time because the goal here is to be direct and help you understand practically how to connect everything.
So, if you're developing a game, app, or anything in Godot that needs to access this Hive information, this tutorial is for you.
What are we going to do here?
In the code I'll provide, we’ll make a query to check if an account exists on the Hive Blockchain using get_profile and get_accounts. Then, if the account is valid, we will fetch the profile details of that account, such as name, ID, reputation, followers, and even the profile picture.
Very simple, but very useful, right?
Result
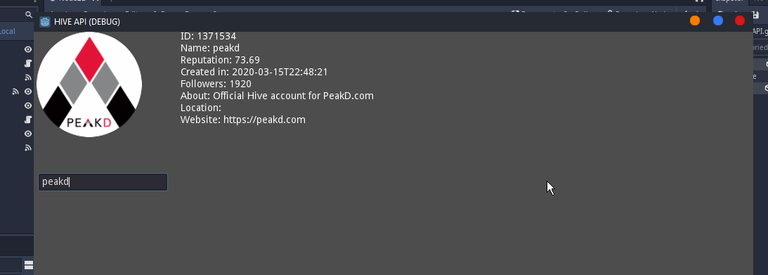
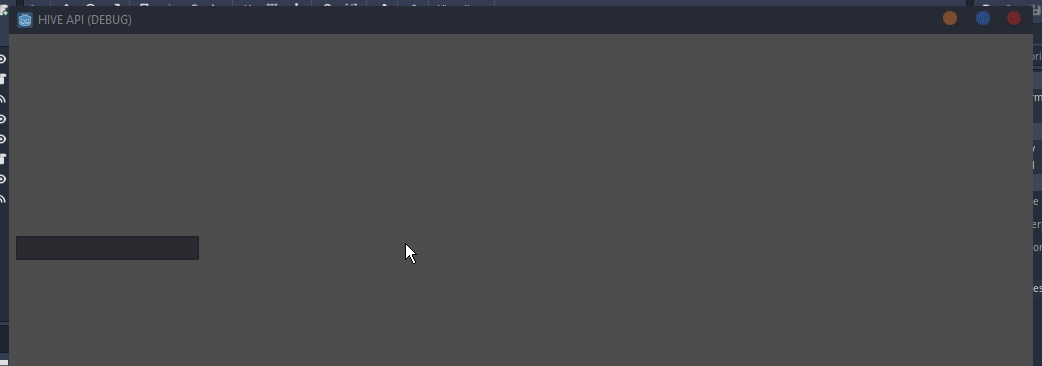
You can create a much better interface than mine, of course. I just kept it simple to check some details that aren’t displayed. It also includes a text box to enter user names and fetch the information.
What we will use
- Node type
Node
orControl
HiveAPI
- LineEdit to be our input box
- Label to display the information
- HTTPRequest (Connected to HiveAPI)
- Node type
Node
orControl
ImageAPI
- TextureRect to display the image
- HTTPRequest2 (Connected to ImageAPI)
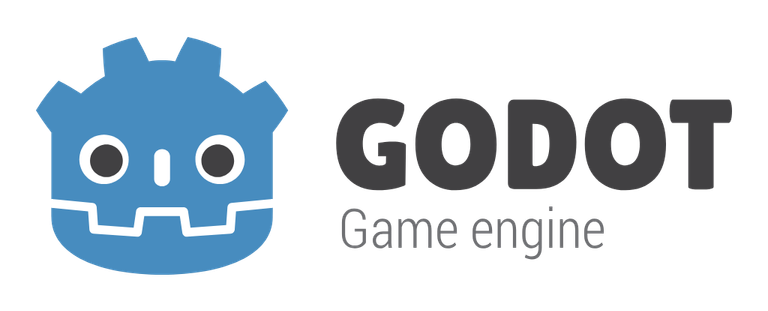
Let’s go to the code!
Here is the script I will explain to you. The idea is to connect Godot to the Hive API using the HTTPRequest
and fetch information for a specific user:
This is the HiveAPI
node, which will be responsible for the main part.
extends Node
# HTTPRequest node
onready var http_request = $HTTPRequest
onready var input_account = $LineEdit
onready var info_label = $Label
var profile_image = ""
var current_request = "" # To track the current request type
func _ready():
http_request.connect("request_completed", self, "_on_request_completed")
# Called when the search button is clicked
func _on_LineEdit_text_entered(new_text):
var account_name = input_account.text.strip_edges()
if account_name == "":
info_label.text = "Please enter a valid account name."
return
# Builds the URL to verify the existence of the user
var url = "https://hivexplorer.com/api/find_accounts?accounts=[%22" + account_name + "%22]"
current_request = "check_user"
if http_request.request(url) != OK:
info_label.text = "Error sending HTTP request."
# Processes the response to requests
func _on_request_completed(result, response_code, headers, body):
if response_code == 200:
var data = parse_json(body.get_string_from_utf8())
if current_request == "check_user":
if data and data.get("accounts") and len(data["accounts"]) > 0:
var account_name = data["accounts"][0]["name"]
# Now requests profile data
request_user_profile(account_name)
else:
info_label.text = "User not found."
elif current_request == "get_profile":
if data:
var id = data.get("id")
var name = data.get("name", "")
var reputation = data.get("reputation", 0.0)
var created = data.get("created", "")
var followers = data.get("stats").get("followers", 0)
var about = data.get("metadata").get("profile").get("about", "")
var location = data.get("metadata").get("profile").get("location", "")
profile_image = data.get("metadata").get("profile").get("profile_image")
var image = get_node("/root/HIVE/ImageAPI")
image.initialize()
var website = data.get("metadata").get("profile").get("website", "")
info_label.text = "ID: %s\nName: %s\nReputation: %.2f\nCreated in: %s\nFollowers: %s\nAbout: %s\nLocation: %s\nWebsite: %s" % [id, name, reputation, created, followers, about, location, website]
else:
info_label.text = "Error processing API data."
else:
info_label.text = "Request error. Code: %d" % response_code
# Makes the request to obtain the user's profile
func request_user_profile(account_name):
var url = "https://hivexplorer.com/api/get_profile?account=%22" + account_name + "%22"
current_request = "get_profile"
if http_request.request(url) != OK:
info_label.text = "Error sending the profile request."
Step-by-Step Explanation
Initializing the HTTPRequest
First, we create an HTTPRequest
and connect it to an event called request_completed
. This event will be triggered every time a request is completed. This is where we will handle the API response.
Search Function (Check if the User Exists)
When the search button is pressed (this happens in the _on_LineEdit_text_entered
function), the script grabs the account name entered by the user and builds the URL to query whether this name exists on the Hive blockchain.
The URL we use is: https://hivexplorer.com/api/find_accounts?accounts=[%22" + account_name + "%22]
.
If the response is successful and the account is found, the function calls another function to fetch the account’s profile details.
Handling the API Response
The request_completed
event handles the response from the API. If the request was successful (response code 200), we try to process the returned data. The first thing we do is check if the user exists.
User Profile Details
When we find the user, we make a new request to fetch the complete profile for that account using the URL: https://hivexplorer.com/api/get_profile?account=%22" + account_name + "%22
.
The response contains several data fields like ID, name, reputation, creation date, followers, location, and even the user’s website. These data are displayed on the Godot interface. I used a profile_image
variable to store the profile image URL, which can be displayed elsewhere in your game or app.
Displaying the Information
All of the data returned by the API is shown on the screen through the info_label
, which displays the detailed information of the user. You can customize this to display the information however you want in your project.
Getting Profile Images
Let’s make a request to fetch the profile image URL for a user, and after obtaining the response, we will display this image in a TextureRect.
This other node I called ImageAPI
will be responsible for fetching and storing the user’s profile images. Remember, to avoid issues with the image format being obtained, you should add:
load_jpg_from_buffer() To fetch JPG images.
load_png_from_buffer() To fetch PNG images.
Hey everyone! Let’s continue with another tutorial that is straightforward and useful for those programming in Godot and wanting to integrate Hive Blockchain account data. If you’re already familiar with the basics of HTTP requests, this code will be an expansion for working with profile images.
Today, we will fetch the profile image of a Hive user using the Hive Explorer API and display it in a TextureRect
in Godot. This approach can be very useful for games or apps that use Hive account data and want to dynamically show profile images.
What are we going to do here?
We will make a request to get the profile image URL for a user, and after obtaining the response, we will display this image in a TextureRect
. This is great if you want to show user profile images in your Godot project.
The code:
Here’s the complete script we’ll explain. Since we’re fetching the image directly from the API and displaying it on the screen, this is a pretty simple integration:
extends Node
# Node references
onready var http_request = $HTTPRequest2
onready var texture_rect = $TextureRect
func initialize():
# URL image
var image = get_node("/root/HIVE/HiveAPI")
var url = image.profile_image
print(url)
# Make the request
var error = http_request.request(url)
if error != OK:
print("Error starting the request:", error)
# Called when the request ends
func _on_HTTPRequest2_request_completed(result, response_code, headers, body):
if result == OK and response_code == 200:
texture_rect.rect_min_size = Vector2(150, 150) # Sets the minimum size (150x150)
texture_rect.stretch_mode = TextureRect.STRETCH_KEEP_ASPECT_CENTERED # Keeps the proportion centered
var image = Image.new()
var err = image.load_png_from_buffer(body) # Try uploading the PNG from
the body
if err == OK:
texture_rect.texture = ImageTexture.new().create_from_image(image) # Creates the texture and applies it to the TextureRect
else:
print("Failed to load the image")
Explanation
Setting Up the Nodes:
This script is attached to aNode
that has anHTTPRequest2
and aTextureRect
(the node where the image will be displayed).Making the Request:
When you press the button to fetch the profile image, it sends a request to the URL of the image. If the request is successful, the image is loaded into theTextureRect
node.Loading the Image:
The profile image is loaded from the API response. After checking if theload_png_from_buffer
function returns OK, we convert the image into a texture and apply it to theTextureRect
in Godot.
In addition, we are adjusting the minimum size of the TextureRect
and setting the stretch option to keep the aspect ratio centered, ensuring that the image is not distorted.
Node structuring
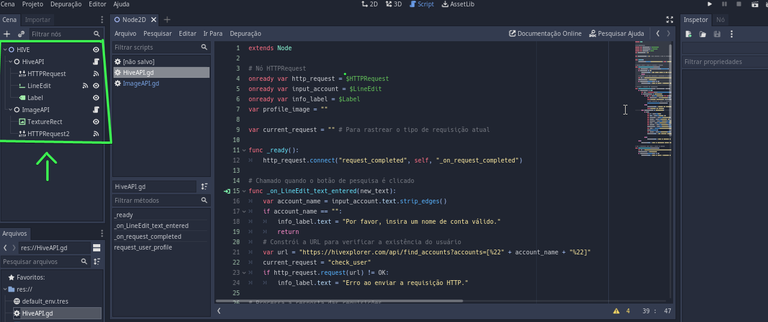
With this simple script, you can already integrate Hive Blockchain data into your Godot project.
Of course, this is just the beginning. You can expand this, get more data, or even use other APIs to enrich your application. Get more interesting information such as transactions, post activity and account votes, communities and more. The beauty of this type of integration is that you can start working with real blockchain data in a super simple and efficient way. This opens up a whole range of possibilities.
I hope this tutorial has been useful to you. If you have any questions, please leave them in the comments.
Published with PeakVault
Congratulations @wlfreitas! You received a personal badge!
You can view your badges on your board and compare yourself to others in the Ranking
Congratulations @wlfreitas! You received a personal badge!
You can view your badges on your board and compare yourself to others in the Ranking
Congratulations @wlfreitas! You received a personal badge!
You can view your badges on your board and compare yourself to others in the Ranking
Check out our last posts: