In this SwiftUI tutorial I will show you how to build an App to change a Logo / Background color via Slider.
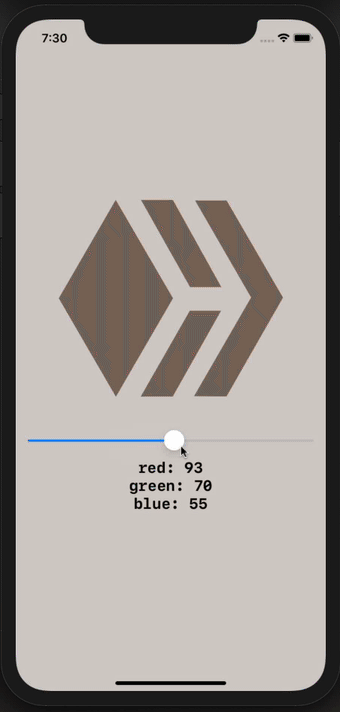
You can find the source code as well on my Github page.
Requirements
- Mac OS
- XCode
- Render As to Template ImageTo change the color of the #Hive Logo you need to upload a vector image to the Assets folder (e.g. pdf) and change
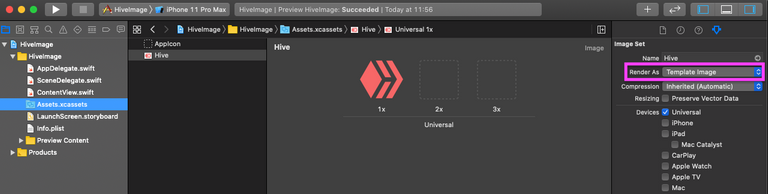
Layout
In SwiftUI you use "Stacks" to align the content on the screen. In this App I use:
- ZStack for showing a background Color
- VStack to display the Image, Slider and Text Vertically to each other.
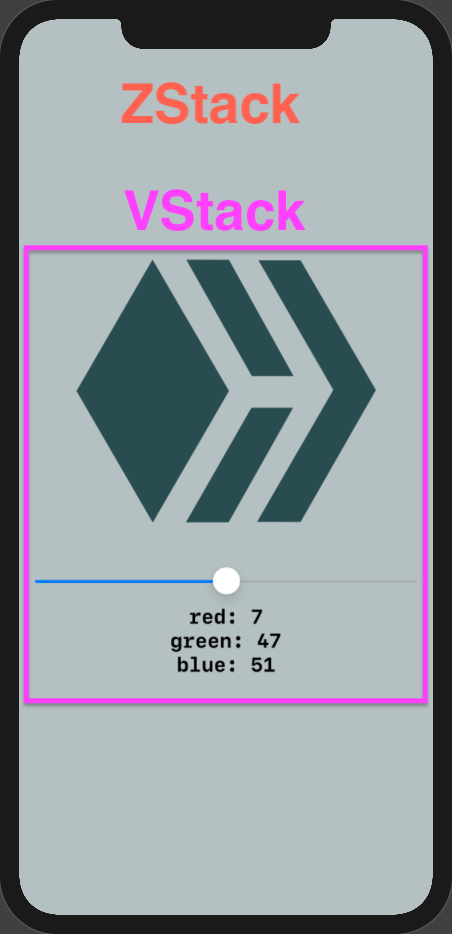
Functionality Overview
- Generate random numbers to be assigned to the red, green and blue value.
- Multiply the slider value with the random color values.
Once the slider is moved, the slider value will be multiplied to each of the different generated color values which causes the color to change anytime the app is started.
Code
- Generate Random Numbers for the red, green and blue value. Notice that that the value 1 is equal to 255.
var redValue = Double.random(in: 0...0.8)
var greenValue = Double.random(in: 0...0.8)
var blueValue = Double.random(in: 0...0.8)
var randomValue = Double.random(in: 0...0.5)
- Set a variable the tracks the State of the Silder. Anytime the slider is moved, the value is updated as well and accessible throughout the same View.
@State private var sliderValue: Double = 0.6
- ZStack is used to show views on top of each other. In this case I use it to set a background color for the app and assign the color values to the numbers generated vis the random number and the Slider.
ZStack {
Color(
red: redValue * sliderValue,
green: greenValue * sliderValue,
blue: blueValue * sliderValue,
opacity: 0.3)
- VStack is used to display the Image, Slider and Text Vertically to each other. You can set a .center attribute to align everything centered.
VStack(alignment: .center) {
Image("Hive")
.resizable()
.scaledToFit()
.frame(width: 300, height: 300, alignment: .center)
.foregroundColor(Color(
red: redValue * (sliderValue + randomValue),
green: greenValue * (sliderValue + randomValue),
blue: blueValue * (sliderValue + randomValue),
opacity: 0.8))
}
- The Slider has the State variable assigned (SliderValue) as start value and can move in the range between 0.2 and 1. I used 0.2 as min value since I multiplicate the values and want to avoid it being 0.
Slider(value: $sliderValue, in: 0.2...1, step: 0.01)
Text("red: \(255 * (redValue * (sliderValue + randomValue)), specifier: "%.0f")")
Text("green: \(255 * (greenValue * (sliderValue + randomValue)), specifier: "%.0f")")
Text("blue: \(255 * (blueValue * (sliderValue + randomValue)), specifier: "%.0f")")
That is the breakdown of the code used for this app.
Please check my my Github page for the entire code or let me know in the comments section if you have questions.