Let's start by creating a model data template with name and role for the Steemit Inc employees using a Struct.
struct Employee {
let name: String
let role: String
}
Now lets create an Array that holds a few of the Steemit.INC (ex)employees .
let steemitInc = [
Employee(name: "Ned", role: "ex-CEO"),
Employee(name: "Vandeberg", role: "Blockchain Developer"),
Employee(name: "Justin", role: "Head of Engineering"),
Employee(name: "roadscape", role: "Senior Product Engineer")
]
To extract all roles from that steemitInc Array we can use the map method in Swift to put all roles into a new array.
var searchRole = steemitInc.map { $0.role }
Finally, having extracted the roles we can now find a user tied to that role using the filter method.
var findUser = steemitInc.filter { $0.role == "ex-CEO"}
The complete Swift Playground looks like this:
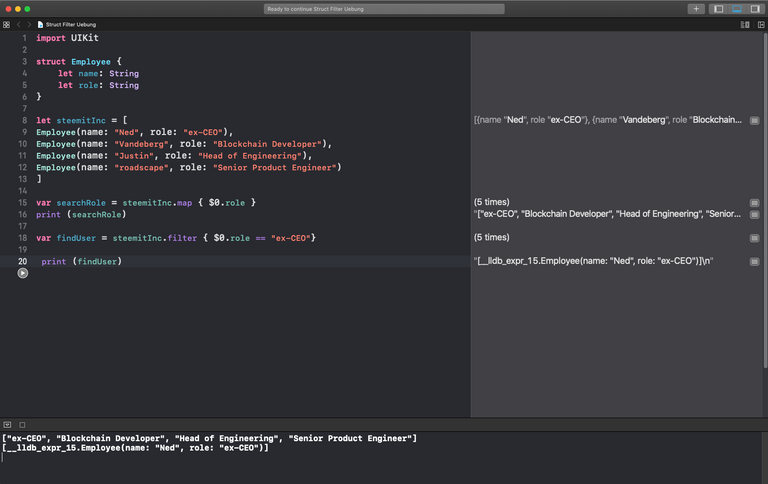
Hopefully you find this little tutorial useful. I linked most of the topics covered to the Apple documentation for further reading.