Introduction
gu-deckcode is an Immutable X officially supported JavaScript library for encoding / decoding Gods Unchained deck codes. This is the tool that is used to create and interpret the deck codes players use regularly, either in the game client or on community websites.
The documentation is outdated in three places, but in the examples below I show how to use the package correctly.
In this post, I will demonstrate its usage through a step-by-step tutorial. These examples were tested in Linux, but they should also work in Windows as they are.
1. Installation
Assuming you already have Node.js and npm installed properly, create a new project folder:
mkdir decks
and get in cd decks/
then install npm i @imtbl/gu-deckcode
.
This should create package.json automatically and a bunch of other necessary files and folders.
2. Importing the module
Create a new file any way you like (I use Visual Studio Code).
If you're going to use CommonJS module syntax, import with
const guDeckcode = require("@imtbl/gu-deckcode");
If you want to use ECMAScript Modules (ESM) syntax instead, import with
import guDeckcode from "@imtbl/gu-deckcode";
3. Finding the library IDs for each card
You will need to provide the library IDs of the cards to create a deckcode. The simplest way to find them is to search this list:
https://api.godsunchained.com/v0/proto?format=flat
4. Encoding decklists
Once you have all the library IDs, put them in an array following this template:
const libraryIds = ["L0-001", "L0-002"];
Take for example this random deck:
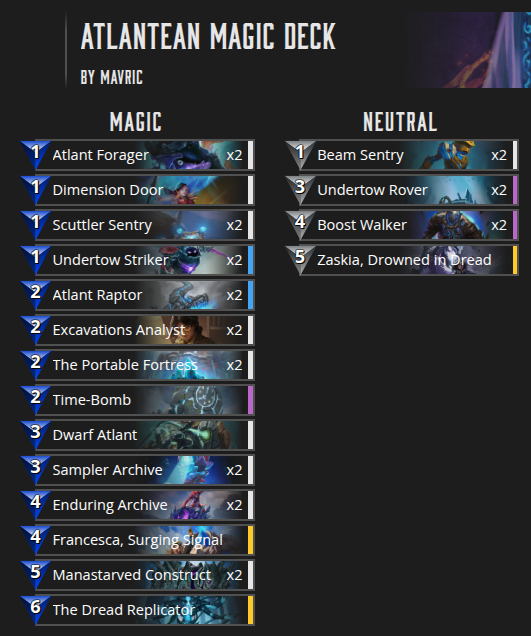
Decklist in GUDecks source
The codes for each card are:
Card Name | Library ID |
---|---|
Atlant Forager | L12-105 |
Dimension Door | L1-362 |
Scuttler Sentry | L10-152 |
Undertow Striker | L12-095 |
Atlant Raptor | L12-097 |
Excavations Analyst | L12-106 |
The Portable Fortress | L2-210 |
Time-Bomb | L2-154 |
Dwarf Atlant | L2-074 |
Sampler Archive | L12-101 |
Enduring Archive | L12-109 |
Francesca, Surging Signal | L12-090 |
Manastarved Construct | L12-110 |
The Dread Replicator | L13-120 |
Beam Sentry | L8-119 |
Undertow Rover | L10-184 |
Boost Walker | L12-001 |
Zaskia, Drowned in Dread | L12-152 |
The corresponding complete array, with duplicates, would be
const libraryIds = ["L12-105", "L12-105", "L1-362", "L10-152", "L10-152", "L12-095", "L12-095", "L12-097", "L12-097", "L12-106", "L12-106", "L2-210", "L2-210", "L2-154", "L2-074", "L12-101", "L12-101", "L12-109", "L12-109", "L12-090", "L12-110", "L12-110", "L13-120", "L8-119", "L8-119", "L10-184", "L10-184", "L12-001", "L12-001", "L12-152"];
A simple working snippet would then be
const guDeckcode = require("@imtbl/gu-deckcode");
const libraryIds = ["L12-105", "L12-105", "L1-362", "L10-152", "L10-152", "L12-095", "L12-095", "L12-097", "L12-097", "L12-106", "L12-106", "L2-210", "L2-210", "L2-154", "L2-074", "L12-101", "L12-101", "L12-109", "L12-109", "L12-090", "L12-110", "L12-110", "L13-120", "L8-119", "L8-119", "L10-184", "L10-184", "L12-001", "L12-001", "L12-152"];
const domain = "magic";
const deckCode = guDeckcode.encode(libraryIds, domain);
console.log(deckCode);
5. Decoding decklists
This is simpler. You grab any decklist code you are interested in, and use it like so
const { libraryIds, domain, formatCode } = guDeckcode.decode("GU_1_4_MCBMCBICPICPBGyKCwKCwMBrMBrMBtMBtMCCMCCCECCECCCyCBWMBxMBxKDcKDcBBDBBDMCFMCFMBmMCGMCGMCwNCQ");
A simple working snippet would then be
const guDeckcode = require("@imtbl/gu-deckcode");
const { libraryIds, domain, formatCode } = guDeckcode.decode("GU_1_4_MCBMCBICPICPBGyKCwKCwMBrMBrMBtMBtMCCMCCCECCECCCyCBWMBxMBxKDcKDcBBDBBDMCFMCFMBmMCGMCGMCwNCQ");
console.log("libraryIds:", libraryIds);
console.log("domain:", domain);
console.log("formatCode:", formatCode);
Which would yield this result
libraryIds: [
'L12-105', 'L12-105', 'L8-119',
'L8-119', 'L1-362', 'L10-152',
'L10-152', 'L12-095', 'L12-095',
'L12-097', 'L12-097', 'L12-106',
'L12-106', 'L2-210', 'L2-210',
'L2-154', 'L2-074', 'L12-101',
'L12-101', 'L10-184', 'L10-184',
'L1-055', 'L1-055', 'L12-109',
'L12-109', 'L12-090', 'L12-110',
'L12-110', 'L12-152', 'L13-120'
]
domain: magic
formatCode: 1
These codes would then need to be mapped back to the JSON output I indicated above to retrieve the card name and a means to display the picture of the framed card, for example.
Format code defaults to 1, corresponding to constructed deck rules. According to the documentation only constructed decks are supported, so you can ignore this setting.
What can you use this for?
It's a simple way to get introduced to JavaScript with a purpose related to something you enjoy, in this case a game. Allows you to learn how to write simple scripts or build dynamic websites based on real data and a concrete example.
Maybe you have a project in mind involving decks, like a database or analysis of matches for example. You want to keep track of the performance of your decks and group similar ones together to aggregate results.
We currently don't have any public tools that analyze a player's performance in great detail and maybe reading this will inspire someone to work on that 😀
Another use case is to build a small tool to keep track of your own cards while a game is going on, so you would always know which cards you have already played and which are still in your deck. Similar tools exist for Hearthstone, for example.
I hope you find this information useful. Thanks for reading!
The rewards earned on this comment will go directly to the people( @agrante ) sharing the post on Reddit as long as they are registered with @poshtoken. Sign up at https://hiveposh.com. Otherwise, rewards go to the author of the blog post.