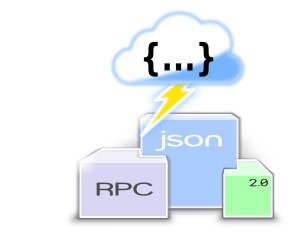
You don't need to use a programming language to access the blockchain. You can use terminal commands or other tools like Rested to create a POST request.
If you have no experience accessing the Steem API, let's go over some basics so you'll be empowered to do great things.
Rested
You can use the Rested Chrome Extension to access the blockchain.
After installing this extension, click on the </>
icon, switch to POST and type in the API endpoint:
Then set the type to Custom
and paste the example jsonrpc request:
The rest of this tutorial assumes you're passing the jsonrpc request using curl
from the terminal.
Terminal
To use this tutorial, we need to access the terminal. Accessing the terminal is outside the scope of this article, but some helpful Google search phrases would be:
how to access the terminal on windows
curl
The command we're going to use is called curl
. It's short for "command line url". Its purpose is to access a web resource pretty much just like a browser, then return the contents of the resource as text.
Installing curl
is also outside the scope of this article. Many operating systems already provide curl
, but some do not. Some can install it with their package manager.
how to install curl on windows
Something Simple: get_account_count
First, let's make our goal simple. We want to know how many accounts there are on the platform. Lucky for us, there's a single API method specifically for doing this, and the method is called condenser_api.get_account_count
.
curl -s --data \
'{
"jsonrpc":"2.0",
"id":1,
"method":"condenser_api.get_account_count",
"params":[]
}' \
https://api.steemit.com
Now, let's go over this command.
curl
- The actual command we're running locally.-s
- Means be silent, don't show statistics of the command. If we leave this off, we'll get some extra data about the request/response cycle ofcurl
, but it's a distraction here.--data
- Tellscurl
we are making a POST request followed by the body of the post in''
single quotes.- POST request body, which is passed by
curl
to the remote host:"jsonrpc"
- Tells the remote host we intend to follow thejsonrpc
spec:"2.0"
"method"
- The full name of the method, in this case"condenser_api.get_account_count"
"params"
- The required parameters of this method, in this case none."id"
- Thejsonrpc
id
that will be repeated to us in the response.
- POST request body, which is passed by
Here's the response, look at the result
value.
{
"id": 1,
"jsonrpc": "2.0",
"result": 1023875
}
The response we get is broken down into the following fields:
"id"
- Thejsonrpc
id
repeated, in this case, we passed1
in the request, so we got1
in the response."jsonrpc"
- Since we asked for"2.0"
, we got the same in the response."result"
- The actual result of the method. At the time of writing, there are 1,023,875 accounts.
Something Slightly Complicated: get_active_votes
The method we're going to try here requires two parameters. When we call condenser_api.get_active_votes
, it needs to know which author
and permlink
we're interested in.
We're going to do the query on my very first post: That's Nutrition!
curl -s --data \
'{
"jsonrpc":"2.0",
"id":1,
"method":"condenser_api.get_active_votes",
"params":["inertia", "that-s-nutrition"],
}' \
https://api.steemit.com
Like before, we have "jsonrpc"
, "id"
, and "method"
. But unlike before, we're not passing an empty "params"
array. Instead, we're passing ["inertia", "that-s-nutrition"]
to identify the exact post we're interested in.
{
"jsonrpc":"2.0",
"result":[
{
"voter":"zebbra2014",
"weight":"5578646898886369",
"rshares":1210323275,
"percent":10000,
"reputation":-2683223274535,
"time":"2016-07-14T01:36:42"
},
{
"voter":"riscadox",
"weight":"400782100556392",
"rshares":86924063,
"percent":10000,
"reputation":"5488837804926",
"time":"2016-07-13T07:34:09"
},
{
"voter":"warplat",
"weight":"321958340660123",
"rshares":69873283,
"percent":10000,
"reputation":"327774469729",
"time":"2016-07-14T02:01:00"
},
{
"voter":"gjhi4552201",
"weight":0,
"rshares":52219629,
"percent":10000,
"reputation":3798781589,
"time":"2016-07-13T07:43:33"
},
{
"voter":"qwertas",
"weight":"68245735399657",
"rshares":75902299,
"percent":10000,
"reputation":-279089549224,
"time":"2016-07-13T07:08:48"
},
{
"voter":"inertia",
"weight":0,
"rshares":240003499,
"percent":10000,
"reputation":"126460782517772",
"time":"2016-07-13T07:02:57"
},
{
"voter":"seb",
"weight":0,
"rshares":110951939,
"percent":10000,
"reputation":"1192866377126",
"time":"2016-07-14T22:12:09"
}
],
"id":1
}
Here, you can see every voter and all of the details that go into their vote. Compare this result to:
https://steemd.com/funny/@inertia/that-s-nutrition
voter
- The person who cast a vote.weight
- The score this vote receives, used by vote payout calculation. Note: Becomes0
if a negative vote or changed votes.rshares
- Shares the voter received as a curation reward.percent
- The integer version of the vote (10000 / 100 = 100.00 %)reputation
- The reputation of the voter now, not when the vote was cast.time
- When the vote was cast.
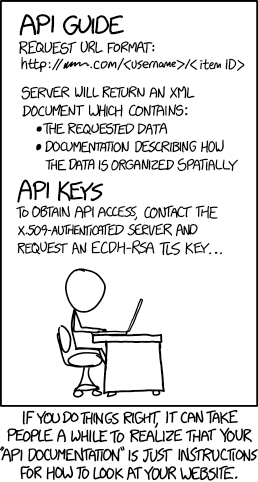
ACCESS LIMITS: Clients may maintain connections to the server for no more than 86,400 seconds per day. If you need additional time, you may contact IERS to file a request for up to one additional second.
Honestly, it's much easier to use a programming language than to construct the jsonrpc requests manually:
Open image in new tab
JSFiddle Shift + Ctrl + J to open JS console.
You're right but some people don't start there, believe me.
I know, but jsfiddle is a convenient way to lure them to the dark side 😃
It's not that much more difficult than using curl, there are even some advantages it has (nothing to install and configure). I, for one, have a love/hate relationship with JSON. The hate part comes mainly from me making a mistake after mistake when writing JSON by hand 😃
Hey man, thanks a lot for this. i'm trying to learn things here and there and this is appreciated! Do more tuts in the future if time allows please :D
There's also:
https://developers.steem.io/tutorials
Awesome, bookmarked. Thanks again!
I think Rested Chrome Extension will even make it a bit tricky the processes seems Complicated, is there a short coming to this method?
Mainly, you have to pass valid JSON, and that's a little tricky. Also, these techniques will not allow you to sign transactions (like cast a vote) unless you have a way to pre-sign them ahead of time.
Wow I guessed so too, I'll take my chances though, thank you for letting me know
It would be quite helpful if one will have the patience in the wait and peruse of the set-up. But believe me, its tedious to learn. Thanks @inertia for sharing. Hope to try it out, because I love learning.
No knowledge is wasted.
One question.
Pls whats the "id" as used up there. Thanks.Thank you @inertia for this post. I was enlightened. I have been coming across it but haven gotten the general idea. Pls it would be appreciated if you throw more light in subsequent times.
If i understood you, an id is a meaningless number that is echoed back to the client on each request. The reason it exists is to simplify the implementation of clients which want to be able to route an incoming response to its initiating request. It also allows distinguishing of push notifications from responses.
Hope that helps
Really appreciated.
The beauty of everything in the blockchain is that all of its operations and transactns are open and transparent. Have a good day my friendThank you so much @inertia.
Hi can you help me some one reported me for something that I didn't do my rep was 44 now it is 0 what can I do about it
nothing you can do nothing.
Dam I can't believe I'm pissed
It doesn't get that way by accident. Let's have a look, shall we?
yep, here's your problem
Not to mention most of your posts are memes whining about this situation and your comments are pretty much the same. But all of them got flagged for plagiarism and spam, and that's pretty much the size of it.
Yeah, that's not gonna work out well for you around here.
Finally found one that wasn't just a stolen photograph without any words on it and a title where you aren't whining about the "unfairness" of being flagged down and blacklisted for lame ass theft...
At least it has words. Tell me again how this is original work?
What do you mean finally found one I've made 100s of original memes 😂😂😂😂🤣
You can get upvotes and your reputation will increase again :)
Thanks 👍
I find very convenient https://github.com/steemit/steem-js that wraps that calls and you get the JSON
Thanks for the useful tips! I am also exploring the APIs but more using Python than direct REST queries
Hmm, the dumbed down version is still mostly above my head. Yet, I know there are many others who will benefit from this and in turn, I will benefit from what they create! :)
Wow great analysis on Steem API, thanks @inertia I was able to learn new stuff from your analysis today, the steem platform is top notch in it dealings!
Wow!!!!
That's a nice content!!!!
Programming on c and c++ is easy than python !!!!
I think it is not easy ..This is so tricky and complicated,,If there have any problem or something missing,Full content will go in vain,,
Thanks for sharing,,
Muy interesante aunque parece algo complicado
Wow that interesting, but the truth is that I do not understand a lot of programming language and those things although I confess @inertia, that if I would like to learn how to program and someday I would like to dedicate the necessary time and learn at least the basics. 😍
I've been exploring the same through Python (not steem-py or piston, just making url requests). This has been a handy reference.
Sweet! Thanks.
@inertia - a fix for using this on the cmd line with windows. Windows handles the quotes differently so use " instead of ' and then escape the " in the json request.
curl --data "{"jsonrpc":"2.0", "id":1, "method":"condenser_api.get_account_count", "params":[]}" https://api.steemit.com
I think Rested Chrome Extension will even make it a bit tricky the processes seems Complicated, is there a short coming to this method?
I just follow you. Have you seen India V/s Ireland Cricket Match At Dublin?