This is fifth part of our Spring Boot application.
- Spring Boot (Introduction) - Part 1
- Spring Boot (Building a web application - Running) - Part 2
- Spring Boot (Building a web application - JPA integration) - Part 3
- Spring Boot (JPA Extended: Master-Detail) - Part 4
- Spring Boot (Introduction for Unit Tests) - Part 5
We have finished the basic stuff of our application. Now we are going to create some unit automated tests.
Before to start coding, let's understand how the unit tests works.
The goal of unit testing is to isolate each part of the program and show that the individual parts are correct.
Usually, the developer is responsible to write unit tests. To write a good test a devoloper should follow some good practices: First, try to test alll operators of an object. Second, set and get values for all attributes of an object and finally, put your object in all possible states.
A test is divided in three steps
- Scenario
- Action
- Validation
Scenario is resposible for create everything that will necessary to run a function.
Action, at this part you have to literally run your function using those objects started on the scenario step.
The last step is the validation, at this one, you have to check if the result of the function, called on action, is exactly what you expect.
That's it, let's start our code.
First, let's create a simple business role for our List Domain.
**The List name cannot be empty.
So, when you call the save method you have to check it out.
@Transactional
@RequestMapping(value = "savelist", method = RequestMethod.POST)
public String save(@RequestParam("name") String name, Model model) {
// Validate Name Error
if (name == null || name.isEmpty()) {
throw new RuntimeException("List name cannot be empty or null");
}
ListDomain list = new ListDomain(name);
repoList.save(list);
return this.lists(model);
}
Now, le'ts create a simple class of test
import static org.junit.Assert.assertTrue;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import org.springframework.ui.Model;
import br.com.namom.todolist.controller.ListController;
import br.com.namom.todolist.persistence.ListRepository;
public class ListControllerTest {
@Mock
ListRepository repoList;
private ListController listContr;
@Mock
Model model;
@Rule
public ExpectedException thrown = ExpectedException.none();
@Before
public void setUp() {
MockitoAnnotations.initMocks(this);
this.listContr = new ListController(repoList);
}
@Test
public void shouldSaveANewList() {
// scenario
String listName = "My New List";
// action
String page = listContr.save(listName, model);
// validation
assertTrue(page.equalsIgnoreCase("mylists"));
}
@Test
public void shouldNotSaveANewListWhenEmptyName() {
// scenario
String listName = "";
thrown.expect(Exception.class);
thrown.expectMessage("List name cannot be empty or null");
// action
listContr.save(listName, model);
}
@Test
public void shouldNotSaveANewListWhenNameIsNull() {
// scenario
String listName = null;
thrown.expect(Exception.class);
thrown.expectMessage("List name cannot be empty or null");
// action
listContr.save(listName, model);
}
One can note that you have to mock some objects before to start your tests. This is necessary because you will not start your application and those objects have to be create.
Another important highlight is the name of the test functions, it should always be as much explained as you need.
Ex: shouldNotSaveANewListWhenNameIsNull
That's it.
Just run the application and you will see it working.
Checkout the full code on my github.
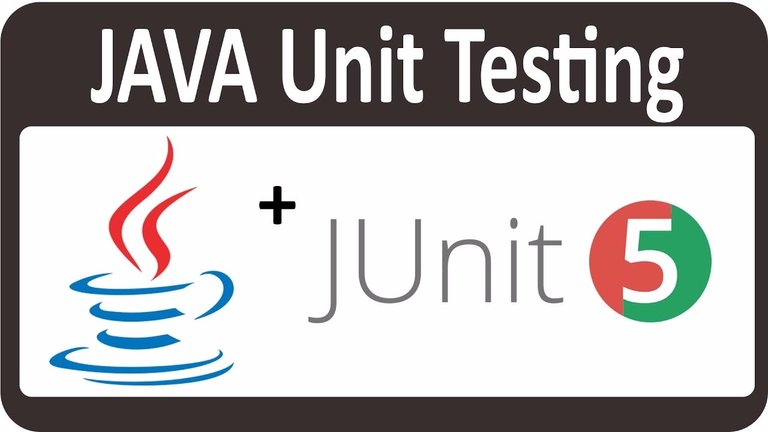
Congratulations @namom! You received a personal award!
You can view your badges on your Steem Board and compare to others on the Steem Ranking
Vote for @Steemitboard as a witness to get one more award and increased upvotes!