This is the fourth part of our Spring Boot application.
- Spring Boot (Introduction) - Part 1
- Spring Boot (Building a web application - Running) - Part 2
- Spring Boot (Building a web application - JPA integration) - Part 3
- Spring Boot (JPA Extended: Master-Detail) - Part 4
- Spring Boot (Building a standalone library) - Part 5
- Spring Boot (Generating a FATJAR) - Part 6
- Spring Boot (Publishing at AWS) - Part 7
At the third part we have built our list and integrated the data-base with JPA/Hibernate. Now we are to create the tasks of a list.
First, lets create our TaskDomain, it is important to understand that which list is going to have as many task as it needs.
import java.io.Serializable;
import java.util.Date;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.ManyToOne;
import lombok.Data;
@Data
@Entity
public class TaskDomain implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Date date;
private Boolean status;
@ManyToOne
@JoinColumn(name = "id_list_domain")
private ListDomain myList;
public TaskDomain(String name, ListDomain mylist) {
this.name = name;
this.date = new Date();
this.status = true;
this.myList = mylist;
}
public TaskDomain() { }
}
On our TaskDomain there is an object called ListDomain and it is noted with @ManyToOne, which means that we are able to have multiples TaskDomain to only one ListDomain. The notation @JoinColumn configures the join attribute between our two domains.
Second, we have to adapt our ListDomain to understand that it can hold a list of Tasks.
import java.io.Serializable;
import java.util.Date;
import java.util.List;
import javax.persistence.CascadeType;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.OneToMany;
import lombok.Data;
@Entity
@Data
public class ListDomain implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private Date date;
@OneToMany(mappedBy = "myList", cascade = CascadeType.ALL)
private Listasd<taskdomain> taks;
public ListDomain(String name) {
this.name = name;
this.date = new Date();
}
public ListDomain() { }
}
Third, lets create our TaskRepository.
import org.springframework.data.jpa.repository.JpaRepository;
import br.com.namom.todolist.domain.TaskDomain;
public interface TaskRepository extends JpaRepository<TaskDomain, Long> {
}
Now we are ready to create our TaskController.
import javax.transaction.Transactional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import br.com.namom.todolist.domain.ListDomain;
import br.com.namom.todolist.domain.TaskDomain;
import br.com.namom.todolist.persistence.ListRepository;
import br.com.namom.todolist.persistence.TaskRepository;
@Controller
public class TaskController {
@Autowired
private TaskRepository taskRepository;
@Autowired
private ListRepository listRepository;
@Transactional
@RequestMapping(value = "/list/{id}", method = RequestMethod.GET)
public String lists(@PathVariable("id") long idList, Model model) {
ListDomain myList = listRepository.getOne(idList);
model.addAttribute("mylist", myList);
model.addAttribute("mytasks", myList.getTaks());
return "list";
}
@Transactional
@RequestMapping(value = "savetask", method = RequestMethod.POST)
public String save(@RequestParam("idList") long idList, @RequestParam("taskName") String taskName, Model model) {
ListDomain myList = listRepository.getOne(idList);
TaskDomain task = new TaskDomain(taskName, myList);
taskRepository.save(task);
return this.lists(idList, model);
}
}
Finally, lets update our list page and create a new page, wich will be resposible for list and save our tasks.
That's it.
Just run the application and you will see it working.
Checkout the full code on my github.
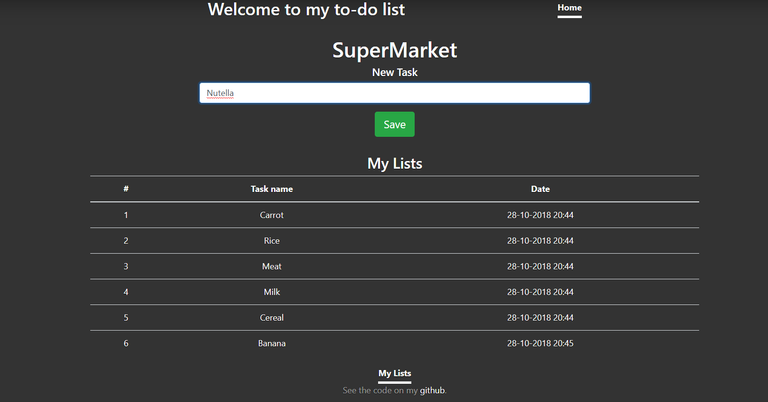