Methods Return
In this article we are going to learn about, returning values of methods..
unlike other programming languages. you can pass many values to a method.. and method can return more than 1 values back to where it was called.
We have seen, our method returning 1 value in previous article. In this article we will look into few methods that return more than 1 values ..
lets take an example..
## Note that in this method, we are returning more than one values
def steemit_fellows
followers = ["bilalhaider", "bago", "ronald", "inertia"]
following = ["abc", "def", "ghi"]
return followers, following
end
puts steemit_fellows
Note: in the above example. we created two Arrays inside of our method. and added few items in them.
and we used single return statement that returned all of the list items. which we displayed using puts statement.
Cooler Method of Return
Do you know, that by default last statement's result of any Ruby Method is returned ..
let see how ..
Note: In this example. last statement of our "calculate_sum" method, didn't used "return" statement,
but when we called the method .. it returned the result.. this happens because ruby by default returns the result of last statement..
Which is Cool :)
def calculate_sum(variable1, variable2)
result = variable1 + variable2
result ## Notice we didn't used return statement
end
puts calculate_sum(100, 2000)
You can also pass unlimited amount of arguments to Ruby methods.
Here we are taking an example of passing variable length arguments to our method.
Example
Take a look at this example. note "*names" allows us to pass variable length arguments to our method.
When we called our method in englishy Style.. we passed to it 3 People.
When we called our method Old fashioned Style.. we passed to it 4 People..
def display_names (*names)
puts "Total number of People are #{names.length}"
for i in 0...names.length
puts "The Person is #{names[i]}"
end
end
### Englishy Style of Calling Methods
display_names "Bilal Haider", "BagoSan Sensei", "David Frank"
### Old Fashioned Styple of Calling Methods
display_names("Walter", "John Ceena", "Kyle", "Adam")
This Skill allows us to write awesome methods. which we can use in our ruby programs..
Key notes
- We learned that our methods can return more than 1 values
- We learned that last statement of our Methods is returned by default
- We learned that we can pass variable length arguments to our methods.
your post all so good. i like it @bilal -haider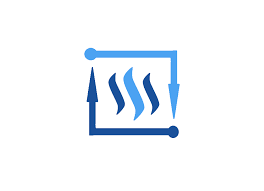
Thanks brother :)
Easy and nice tutorial, great post bro
I am glad you liked my post :)