Today, I will be making a tutorial on how you can make a php captcha with a pronounceable text using php gd2. Some of the time , the words in a captcha are not pronounceable , so users get to make a lot of mistakes filling the forms.
This time, I would try to use a method to make the captcha more readable.
Before we start the tutorial, we would be using php extension called php gd, so it's imperative that that extension is activated in php installation.
Make sure the red-boxed area is ticked.
You could also try editing the php.ini file by making sure it is not commented out as shown below:
Just make sure there is no semi colon (;
) behind it.
These are the steps/procedures below
Create a file called 'captcha.php'.
Insert codes below to start a session
<?php
//Start A session to create to allow use of $_SESSION variables
session_start();
//Create a unique string using the time string
$_SESSION['count'] = time();
Create the Captcha Image
$image = imagecreatetruecolor(200, 50) or die("Cannot Initialize new GD image stream");
$background_color = imagecolorallocate($image, 255, 255, 255);
$text_color = imagecolorallocate($image, 0, 255, 255); //Create Color for text
$line_color = imagecolorallocate($image, 90, 64, 64); //Create the color for line
$pixel_color = imagecolorallocate($image, 10, 70, 255); //create color for pixel
imagefilledrectangle($image, 0, 0, 200, 50, $background_color); create reectangle 50 by 200
Create two Crossing Line
for ($i = 0; $i < 3; $i++) {
imageline($image, 0, rand() % 50, 200, rand() % 50, $line_color);
}
Create pixel dots , about 1000 of it
for ($i = 0; $i < 1000; $i++) {
imagesetpixel($image, rand() % 200, rand() % 50, $pixel_color);
}
Create Readable texts by dividing it into four and putting a kind of prefix, root , suffix and an extra suffix , just like most english words are formed., the array can be further filled
$prefix = array('bl','pr','ok','pl','dr','pr','in','cr','fr','d');
$root = array('a','e','i','o','u');
$suffix = array('ck','gl','t','k','cl','b','n','m','k','l');
$uSuffix = array('ation','ition','able','agia','or');
Radomise the Array Picks , we have to use a -1 becuase arrays start from 0
$pNum = rand(0,count($prefix)-1);
$rNum = rand(0,count($root)-1);
$sNum = rand(0,count($suffix)-1);
$uNum = rand(0,count($uSuffix)-1);
create word
$word = $prefix[$pNum].$root[$rNum].$suffix[$sNum].$uSuffix[$uNum];
Create Color
$text_color = imagecolorallocate($image, 0, 0, 0);
Create Image with a randomised positioning and a text file (arial.ttf)
imagettftext($image, 20, 0, rand(10,90), rand(20,45), $text_color, 'arial.ttf', $word);
Create Image
header("Content-type: image/png");
imagepng($image);
Close the php tag and save the file
?>
By now, running this file should give you something like this and when reloaded the text should change as shown below.
So, next is we create the form page that will use the captcha image.
Create a file and name it form.php
Insert the codes below
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" enctype="multipart/form-data">
<p><input type="text" name="name" /></p>
<img src="captcha.php"/>
<p><input type="text" name="code" /></p>
<p><input type="reset" name="submit" value="Send" /></p>
</form
Insert the php codes to process for the form. this should be placed above the form
<?php
//Start a sessio to have access to session variables
session_start();
//Processing forms by validating via $_POST['submit']
if(isset($_POST['submit'])) {
//Name and Captcha was sent
if(!empty($_POST['name'])) && !empty($_POST['code'])) {
//If sent code is equal to the captcha code
if($_POST['code'] == $_SESSION['rand_code']) {
// send email
$accept = "Thank you for contacting me.";
} else {
//Error Generation when there is a wrong code
$error = "Please verify that you typed in the correct code.";
}
}
//Checking if all form fields were filled
else {
$error = "Please fill out the entire form.";
}
}
?>
So, after this, we'll be having two php files:
Captcha.php
<?php
//Start A session to create to allow use of $_SESSION variables
session_start();
//Create a unique string using the time string
$_SESSION['count'] = time();
$image = imagecreatetruecolor(200, 50) or die("Cannot Initialize new GD image stream");
$background_color = imagecolorallocate($image, 255, 255, 255);
$text_color = imagecolorallocate($image, 0, 255, 255); //Create Color for text
$line_color = imagecolorallocate($image, 90, 64, 64); //Create the color for line
$pixel_color = imagecolorallocate($image, 10, 70, 255); //create color for pixel
imagefilledrectangle($image, 0, 0, 200, 50, $background_color);// create reectangle 50 by 200
for ($i = 0; $i < 3; $i++) {
imageline($image, 0, rand() % 50, 200, rand() % 50, $line_color);
}
for ($i = 0; $i < 1000; $i++) {
imagesetpixel($image, rand() % 200, rand() % 50, $pixel_color);
}
$prefix = array('bl','pr','ok','pl','dr','pr','in','cr','fr','d');
$root = array('a','e','i','o','u');
$suffix = array('ck','gl','t','k','cl','b','n','m','k','l');
$uSuffix = array('ation','ition','able','agia','or');
$pNum = rand(0,count($prefix)-1);
$rNum = rand(0,count($root)-1);
$sNum = rand(0,count($suffix)-1);
$uNum = rand(0,count($uSuffix)-1);
$word = $prefix[$pNum].$root[$rNum].$suffix[$sNum].$uSuffix[$uNum];
$text_color = imagecolorallocate($image, 0, 0, 0);
imagettftext($image, 20, 0, rand(10,80), rand(20,45), $text_color, 'arial.ttf', $word);
imagepng($image,'a.png');
?>
Form.php
<?php
include ('captcha.php');
//Processing forms by validating via $_POST['submit']
if(isset($_POST['submit'])) {
//Name and Captcha was sent
if(!empty($_POST['name']) && !empty($_POST['code'])) {
//If sent code is equal to the captcha code
if($_POST['code'] == $_SESSION['rand_code']) {
// send email
$accept = "Thank you for contacting me.";
} else {
//Error Generation when there is a wrong code
$error = "Please verify that you typed in the correct code.";
}
}
//Checking if all form fields were filled
else {
$error = "Please fill out the entire form.";
}
}
?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post" enctype="multipart/form-data">
<p><input type="text" name="name" /></p>
<img src="a.png"/>
<p><input type="text" name="code" /></p>
<p><input type="reset" name="submit" value="Send" /></p>
</form>
Apart from this, we will also need to have a font file(arial.ttf
) in your directory because it was used while creating the captcha image (imagettftext($image, 20, 0, rand(10,90), rand(20,45), $text_color, 'arial.ttf', $word);
). You can simply search and download that from the internet.
Apart from this, we will also need to have a font file(arial.ttf
) in your directory because it was used while creating the captcha image (imagettftext($image, 20, 0, rand(10,90), rand(20,45), $text_color, 'arial.ttf', $word);
). You can simply search and download that from the internet.
So, now , just go to http://localhost/folder/form.php on ypur local server if that is what you are using to test these codes. Feel free to ask any question in the comments.
After this is done , your form,php
should look like this below.More so, take note that you are free to add your own styles, the form.php have no styling, it's just for the sake of the tutorial
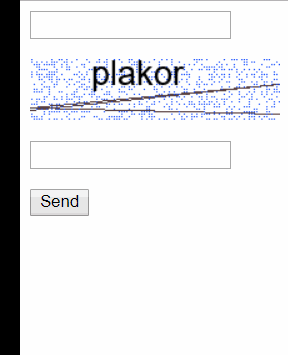
So, now , just go to http://localhost/folder/form.php on ypur local server if that is what you are using to test these codes. Feel free to ask any question in the comments.
Posted on Utopian.io - Rewarding Open Source Contributors
Very nice write up!!! Keep it up, our community needs you
Thanks
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Good
Thnaks
Hey @akintunde I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x