'Special effects' is a general term because, although it might immediately make you think of explosions and AT-AT Walkers, we need to start off much simpler. So, our very first special effect is going to be taking an image and converting it to grey-scale. This will introduce you to the core special effects function, imagefilter().
Step 1. How To Apply Special Effects
There are several different effects you can get out of imagefilter() if you know the magic parameters to pass to it. Each effect requires different kinds of parameters to be passed to it, which is a shame as it means you need to be switched on when using the function. Some effects, such as grey-scale, require no parameters but, even then, it seems like a function such as 'imagegrayscale()' (note the American spelling) would be much easier.
<?php
$image = imagecreatefrompng('frozen-bubble.png');
if (imagefilter($image, IMG_FILTER_GRAYSCALE))
{
echo "Image converted successfully.\n"; imagepng($image, 'frozen-bubble-grey.png');
}
else
{ echo "Image conversion failed.\n"; } imagedestroy($image);
?>
The parameters for imagefilter() are an image resource file, constant to choose the filter effect you want, then three varying arguments. The second parameter is what determines which effect is to be performed. Let's take a look at grey-scaling images:
The code doesn't include error checking to ensure $image was loaded properly, so add it, or make sure that frozen-bubble.png (or whatever picture file you're working with) is in the same directory as the script. Save the script as fx1.php and run it from the command line. Another filter closely related to grey-scaling is colourising, which adds to or subtracts from the individual red, green and blue values in each of the pixels in your image. This is where the three extra parameters come in: parameter three specifies the red variation, four does green and five does blue. It's important to note that it adds to or subtracts from the existing colour, which means that specifying 0 means 'no change to this colour'. This is an example that will make the output picture look more red than usual:
<?php
$image = imagecreatefrompng('frozen-bubble.png');
if (imagefilter($image, IMG_FILTER_COLORIZE, 150, 0, 0))
{
echo "Image converted successfully.\n";
imagepng($image, 'frozen-bubble-colourised.png');
}
else
{
echo "Image conversion failed.\n"; } imagedestroy($image);
?>
As you can see, it's largely the same as grey-scaling, with the addition of the extra parameters. As a result, we'll only print the imagefilter() line where the rest of the script is the same as above. If you want to subtract colours from an image, just use negative numbers. For example, this call to imagefilter() will take out all red and green in the image:
if (imagefilter($image, IMG_FILTER_COLORIZE, - 255, -255, 0)) {
So, we have two conditions in our 'if' statement: if imagefilter() grey-scale worked and imagefilter() colourisation worked. Now, as I said, PHP will execute these in left-to-right order, which means it will do what we want – convert to grey-scale and add colours. The colours we've added are 50 red and 10 green, which should give a warm sepia tone.
Step 2: How to Apply Creative Effects
Quite a few of the effects you can use are subtle but, when used correctly, make your pictures look better. Our favourite is the Gaussian blur filter, which does a great job of making images look ever-so-slightly out of focus. Annoyingly, the PHP implementation doesn't have any way to control the strength of the Gaussian blur used on the picture, so its use is quite simple:
if (imagefilter($image, IMG_FILTER_GAUSSIAN_ BLUR)) {
Altering the brightness and contrast are probably the most common image operations around, and they both work in the same way by providing an extra parameter between 255 and -255 for the adjustment. The negative numbers decrease brightness yet increase contrast, so be careful how you use them. The effect from these functions are both particularly good – chances are you'll find that a bit of brightness and contrast (about 20 to 30) should be added to most pictures from digital cameras (particularly those shot inside) just to give them a bit more life. This is brightness in action:
if (imagefilter($image, IMG_FILTER_BRIGHTNESS, 100)) {
And if you're looking for a catch-all, 'make livelier' command, you could try this:
if (imagefilter($image, IMG_FILTER_BRIGHTNESS, 20) && imagefilter($image, IMG_FILTER_CONTRAST, -20)) {
Step 3: How to Apply Crazy Effects
OK, so this is where all the fun bits are: effects that don't add realism, and they don't add quality either. Instead, they're destructive, but if you're careful how you use them – and if you're of an artistic persuasion – they can really add some style to your work.
First up is perhaps the filter that's hardest to use in terms of getting output you're happy with: mean removal. This filter can be considered as 'extreme sharpening' – each pixel takes part of the value of the eight pixels surrounding it, inverts the average of that and then multiplies its own value by nine and sums the two. So, in effect, this filter makes the difference between each pixel on the screen much greater – much more than normal sharpening would, which is why the filter is quite hard to use! Here it is in PHP:
if (imagefilter($image, IMG_FILTER_MEAN_ REMOVAL))
You think it looks a bit too simple? Perhaps, but the final output is a different kettle of fish, particularly for the Frozen Bubble picture! Another 'crazy but fun' filter to play around with is edge detection, although this actually works out much easier to use in terms of getting good output. As before, it's called without parameters, so the code is just:
if (imagefilter($image, IMG_FILTER_EDGEDETECT)) {
This outputs an image that looks like it's been heavily embossed before being painted over with metallic colours. Although there are lots of uses for edge detection, our favourite is for caricatures, which is the subtle art of taking a photograph and making it look more like a cartoon. This is best combined with the imagetruecolortopalette() function, so that it converts the image to 16 colours (or something similar) beforehand, otherwise your attempts to fill in-between the edge-detected lines will likely fail owing to colour variation.
The final two special effects I want to look at are embossing and colour reversing, both of which require no extra parameters:
if (imagefilter($image, IMG_FILTER_EMBOSS)) {
And negation, the very similar:
if (imagefilter($image, IMG_FILTER_NEGATE)) {
As I discussed earlier, the embossing filter is quite like edge detection, but is a great deal less pronounced. Colour reversing (IMG_FILTER_NEGATE) is often referred to as 'solarise', but it just flips each colour for its direct opposite, as you would see if you looked at a 35mm film negative.
This wraps up our special effects coverage for this month. As we've seen together, most of these 'special effects' can be achieved with just the imagefilter() function (and further enhanced with a touch of artistic flair and natural talent), and it's extremely versatile, as long as you remember all the parameters it takes. Perhaps in the future, we'll come across functions like imagegrayscale() and imagecolorize(), but let's not hold our breath!
Step 4: How to Make Your Own Filters!
The coding isn't tricky, as long as you know how it's done.
The imagefilter() function in PHP works wonders, but in terms of improving a plain image, it's limited. However, it's simple to write your own filters using PHP code. You'll need to use the imagesx() and imagesy() functions to get the X and Y size of the picture, then loop through each pixel applying your transformation using imagesetpixel() to update the values. For example, you could write a blur filter in PHP by looping through each pixel and setting up an array of the colours of all the surrounding pixels. This should be averaged to give an average value, and use that as the new colour value for the pixel. You can change the blur by sampling pixels further away from the source pixel. Using pixels one place away from the source pixel gives a gentle blur; using pixels 10 places away creates a large blur. This would lead to code along these lines:
dist = 4;
for ($x = 0; $x < $imagex; ++$x) {
for ($y = 0; $y < $imagey; ++$y)
{
for ($k = $x - $dist; $k
<=$x + $dist; ++$k)
{ for ($l = $y - $dist; $l <= $y + $dist; ++$l) {
}
}
}
}
Yes, having four for loops isn't likely to execute quickly, but if you want flexibility and speed, your only choice is to write it in C.
Posted on Utopian.io - Rewarding Open Source Contributors
Your contribution cannot be approved yet because it is not as informative as other contributions. See the Utopian Rules. Please edit your contribution and add try to improve the length and detail of your contribution (or add more images/mockups/screenshots), to reapply for approval.
You may edit your post here, as shown below:
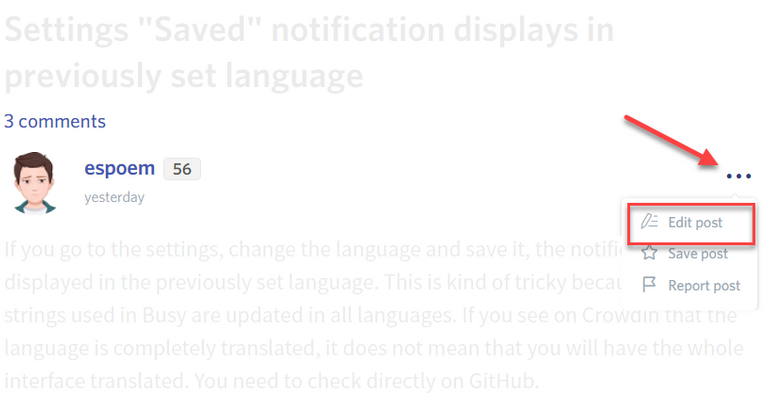
You can contact us on Discord.
[utopian-moderator]
Fixed. Kindly check again.
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @alv I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x