What Will I Learn?
- Create menu with data verification
- Using the while loop
- Development of a 4 operations calculator
Requirements
- Basic knowledge of programming
- Have Python 3.6.4 installed
- Have Sublime Text or Ninja-IDE installed (optional)
Difficulty
- Intermediate
Tutorial Contents
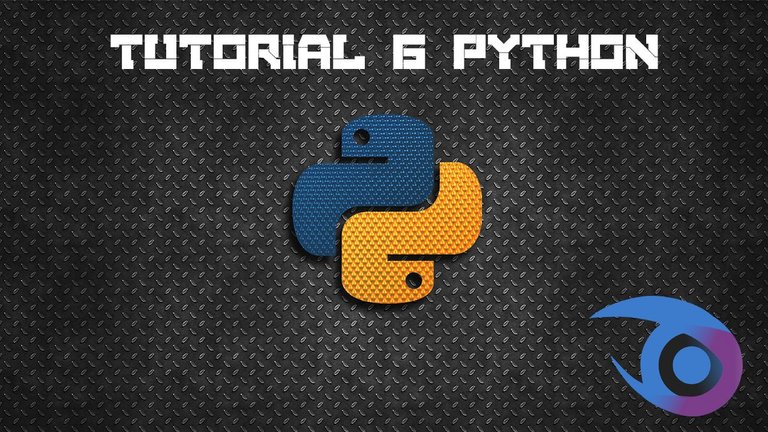
Fuente
Welcome to this new tutorial in Python, we will see how to make a program with all the tools learned previously, and we will use one of the most used cycles in programming, we will learn how to make a menu with options, in this case it will be about a calculator with the 4 basic operations: add, subtract, multiply and divide, as we can verify input data and exit the program completely.
I recommend you go through the previous tutorials since it will be understood that you have practiced the previous exercises. As we already know, this program will try to make a calculator with the 4 operations, but we will design a menu for the user to enter the operation and the numbers that he wants to use, and we will keep it within the program until he decides the moment indicated to get out, for this we will use some of the cycles called "while" but first design the menu, I must clarify that in my case I use "Windows" if you use another operating system certain system commands change, that would be the beginning to create the menu:
# Calculator
import os
w = "Welcome to the calculator"
option = 6
print (w.center(50, "="))
print ("Select which operation you want to perform")
print ("1 to add numbers")
print ("2 to subtract numbers")
print ("3 to multiply numbers")
print ("4 to divide numbers")
print ("5 to exit the program")
If we execute the program we realize that it simply throws us a complete menu, but it is necessary to ask the user which option to choose, we must save in a variable a number between 1 to 5, making sure that if you enter a number outside this range, you will an error and re-enter the correct data, for this we use the "while" cycle, which will allow us to repeat the times we want to show the instruction until a certain condition is met, in the following code we can see how to use it in this case:
while option > 5: #Verification that the user chooses a valid option
option = int(input('Select an option from 1 to 5: '))
if option > 5 or option < 1:
print ("Enter a correct option (1-5)")
This is the reason why before the menu we create a variable and assign it in this case the value of "6" if the user enters this number or a different one to the range it will repeat the error message several times until he simply chooses the correct option , now we will do the respective operations for each option of our program, for this we will use the conditionals if, elif and else, they will allow us to repeat again other operations and to leave the program completely, we will see the codes separately and then we join them all in a single page of the code to compile the program.
In the case of a sum the following code would be:
if option == 1: #to add numbers
os.system('cls')
print ("Sum of Numbers".center(50, " "))
a = int(input('Enter the first number: '))
b = int(input('Enter the second number: '))
add = a + b
print ("The result is:", add)
R = input('Do you want to perform another operation?: y/n ')
if R == "y":
option = 6
os.system('cls')
elif R == "n":
os.system('exit')
For the subtraction it would be very similar to the sum we just have to change the symbol "+" for "-" as the multiplication we change it for "*" as they are very similar, I will place them in a single block:
elif option == 2: #Subtract numbers
os.system('cls')
print ("Subtraction of numbers".center(50, " "))
a = int(input('Enter the first number: '))
b = int(input('Enter the second number: '))
sub = a - b
print ("The result is:", sub)
R = input('Do you want to perform another operation?: y/n ')
if R == "y":
option = 6
os.system('cls')
else:
os.system('exit')
elif option == 3: #multiply numbers
os.system('cls')
print ("Multiplication of numbers".center(50, " "))
a = int(input('Enter the first number: '))
b = int(input('Enter the second number: '))
mul = a * b
print ("The result is:", mul)
R = input('Do you want to perform another operation?: y/n ')
if R == "y":
option = 6
os.system('cls')
else:
os.system('exit')
In the case of the division we must check that the divisor is a totally different number of "0" so we must make a check throwing an error that can not live a number between zero, for that we apply the following code:
elif option == 4: #Divide numbers
b = 0
os.system('cls')
print ("Division of numbers".center(50, " "))
a = int(input('Enter the first number: '))
while b == 0: #Data check
b = int(input('Enter the second number: '))
if b == 0:
print ("You can not divide by 0, try again with another number")
div = a / b
print ("The result is:", div)
R = input('Do you want to perform another operation?: y/n ')
if R == "y":
option = 6
os.system('cls')
else:
os.system('exit')
Finally we apply some commands so that the user leaves the program completely if he selects the corresponding option, we will do it in the following way:
elif option == 5: #Exit the program
R = input('Do you really want to leave the program?: y/n ')
if R == "y":
os.system('exit')
else:
os.system('cls')
option = 6
If you notice in the blocks of the codes the variable "option" equals 6, this is a technique that often so that the program repeats itself from the beginning, for this we added a "while" from the beginning. understand that as long as "option" has a value equal to 6, it assures us that it repeats the whole process, so if the user wishes to do another operation, he returns it to the main menu, the final code would be as follows:
# Calculator
import os
w = "Welcome to the calculator"
option = 6
while option == 6: #Program menu
print (w.center(50, "="))
print ("Select which operation you want to perform")
print ("1 to add numbers")
print ("2 to subtract numbers")
print ("3 to multiply numbers")
print ("4 to divide numbers")
print ("5 to exit the program")
while option > 5: #Verification that the user chooses a valid option
option = int(input('Select an option from 1 to 5: '))
if option > 5 or option < 1:
print ("Enter a correct option (1-5)")
if option == 1: #to add numbers
os.system('cls')
print ("Sum of Numbers".center(50, " "))
a = int(input('Enter the first number: '))
b = int(input('Enter the second number: '))
add = a + b
print ("The result is:", add)
R = input('Do you want to perform another operation?: y/n ')
if R == "y":
option = 6
os.system('cls')
elif R == "n":
os.system('exit')
elif option == 2: #Subtract numbers
os.system('cls')
print ("Subtraction of numbers".center(50, " "))
a = int(input('Enter the first number: '))
b = int(input('Enter the second number: '))
sub = a - b
print ("The result is:", sub)
R = input('Do you want to perform another operation?: y/n ')
if R == "y":
option = 6
os.system('cls')
else:
os.system('exit')
elif option == 3: #multiply numbers
os.system('cls')
print ("Multiplication of numbers".center(50, " "))
a = int(input('Enter the first number: '))
b = int(input('Enter the second number: '))
mul = a * b
print ("The result is:", mul)
R = input('Do you want to perform another operation?: y/n ')
if R == "y":
option = 6
os.system('cls')
else:
os.system('exit')
elif option == 4: #Divide numbers
b = 0
os.system('cls')
print ("Division of numbers".center(50, " "))
a = int(input('Enter the first number: '))
while b == 0: #Data check
b = int(input('Enter the second number: '))
if b == 0:
print ("You can not divide by 0, try again with another number")
div = a / b
print ("The result is:", div)
R = input('Do you want to perform another operation?: y/n ')
if R == "y":
option = 6
os.system('cls')
else:
os.system('exit')
elif option == 5: #Exit the program
R = input('Do you really want to leave the program?: y/n ')
if R == "y":
os.system('exit')
else:
os.system('cls')
option = 6
In this way we apply all the knowledge learned in the previous tutorials and additionally we learn how to use one of the most used cycles in programming specifically Python with a calculator of basic operations and checks of some data, if you have any questions use the comments section, glad to help. See you at the next one!
Curriculum
Place here a list of related tutorials you have already shared on Utopian that make up a Course Curriculum, if applicable.
- Tutorial #1 Python: Declaración de variables y Tipo de datos
- Tutorial #2 Python: Comentarios y Operadores Aritméticos
- Tutorial #3 Python: Tuplas y Listas
- Tutorial #4 Python: Diccionarios y Operadores Racionales
- Tutorial #5 Python: Operadores lógicos y Control de flujo.
Posted on Utopian.io - Rewarding Open Source Contributors
Hey! I just upvoted you. Nice post! BTW.
Thanks for your support!!
Thank you for tutorial. :)
no conocosco del tema pero se ve que le pusiste dedicacion gracias
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Buen post se ve que sabes mucho acerca del tema, te felicito la verdad es interesante aprender de los demas tienes mi voto @gabox exitos
Hey @gabox I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x