What Will I Learn?
- The user will learn the concept of JColorChooser in Java programming
- The user will learn how to create a program that implements the concept of JColorChooser.
Requirements
- A fully functional computer system is required for this tutorial
- On the computer system, Java Development Kit (JDK) must be installed.
- Also the computer system must have Java IDE installed. Probably Eclipse.
Get JDK here
Get Eclipse IDE here
Difficulty
- Intermediate.
Tutorial Contents
Welcome guys, this happens to be my third tutorial and we atre going to continue on Graphics related stuffs in Java. The last tutorial has to do with drawing graphics in Java Programming and today, we are looking at the concept of JColorChooser.
JColorChooser
A Color Chooser is an API or better still a component that helps a user in choosing from a pallet of color in a Graphical User Interface environment within a Java program. JColorChooser is a class in Java programming that is found within the swing.
In graphics related programs, there might be a need for a user to select preferred color while doing some stuffs with our Java program, and so JColorChooser comes in play.
This tutorial will help us in understanding the concept of JColorChooser with JFrame. We will be creating a Java program that implement this aspect. To get this done, I will be explaining every line of code as we proceed in creating this program. This will help us in understanding this concept pretty quickly.
The program will have multiple classes with tee.java
being the subclass and king.java
being the main class for the programmer. Do not mind me, I love using my name for classes and sometimes variables as well. So let's get on it guys.
The first class is going to be our sub class tee.java
and we are starting with it right away.
Sub-class tee.java
Like I said, this happens to be our first class. The below screenshots show how our sub-classs tee.java
looks after this tutorial. You can take a quick glance at it.
Now that you have taken a look, we will start with it right away
Block code for tee.java
Firstly, we will need to import the clases needed for this program. To do this, we make use of the import statement as used below.
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
Those classes imported above is used in creating our frame and also prepare us to use the JColorChooser
which is the main focus of this tutorial. Like I mentioned earlier, the JColorChooser
is found within the swing class. Since user will be taking some actions (ie choosing color) in this program, then there will surely be some events taking place and ActionListener will also be implemented in this program. Hence, we need to import the classes needed for us to be able to implement EventHandling and ActionListening which are found in AWT class. So we imported java.awt*
.
That's that about our import
statements. The next thing we need to do is to extend our tee
class and make it look like it does below.
public class tee extends JFrame{
You could see we have extended our tee
class. The extends JFrame
added to this class pretty much let us use our frame as needed in the program.
The next thing we need to do is to declare our components needed in this program
private JButton b;
private Color c = (Color.WHITE);
private JPanel p;
We have declared three components; JButton b
, Color c
and JPanel p`` and they are now ready to be called upon (used) within the program.
Now we need to create a method for the class tee
and inside it is where we will have our stuffs.
public tee(){
Since it is a method, it will not take any parameter. That done, let's start putting our stuffs in there.
Let's create the set title for our frame.
super("Color Chooser Program");
We set the title as Color Chooser Program
.
Next is to create a JPanel component and also set background color for the panel. Also we will have to add our button component to our frame. Remember we already declared these components above, so we just need to implement them here. See how we do that below.
p = new JPanel();
p.setBackground(c);
b = new JButton("Click to choose your color");
As you can see, we have created a new JPanel
and set the background color as the default or initial color we set when declaring our components - Color.WHITE
. Above, we have also added a button with String of texts ``"Click to choose your color"`.
Now the next thing we need to do is to create some ActionListening
and EventHandling
. These will be responsible for ensuring that a user can perform some actions (ie clicking a particular button to choose colors). As the ActionListener
await actions to take place, the EventHandler
will handle (take care) of the events that happen. Let's do that now.
b.addActionListener(
new ActionListener(){
public void actionPerformed(ActionEvent event){
c = JColorChooser.showDialog(null, "Pick your color", c);
We declare action listening for the JButton b
and that makes the program to be awaiting an action on the button (ie when the button is pressed). When the button is pressed, the JColorChooser
dialog pop up with the string title "Pick your color"
and thereby handling the event. There, the user can choose the color he wishes to use. The JColorChooser.showDialog
takes three parameters. The first parameter usually take null
, the second parameter represents the title of the ColorChooser window and the last parameter is the initial color selected.
Now we need to consider if a user doesn't choose a color at all. And for this, we are going to set some conditions using the if
statement.
if(c==null)
c=(Color.WHITE);
With the above lines of code, if the color a user choses is nothing, (ie null
), then we want the color to remain the initial color which is WHITE
.
The next thing we are going to do after that is to set the background color.
p.setBackground(c);
Now that we have done that, the next thing we are going to do is to add our components; panel and button to the frame. To do that, we use the following lines of code.
add(p, BorderLayout.CENTER);
add(b, BorderLayout.SOUTH);
That pretty much put our components at the selected positions.
Now we are going to add the last lines of code for our sub-class tee.java
.
setSize(450,200);
setVisible(true);
These lines of code will be used to set our frame size and also set the frame visibility to true
. That is all for our sub-class tee.java
. We will create another class which will be our main class. But before going into that, let us take a look at the full code for sub-class tee.java
below.
tee.java Full Code
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class tee extends JFrame{
private JButton b;
private Color c = (Color.WHITE);
private JPanel p;
public tee(){
super("Color Chooser Program");
p = new JPanel();
p.setBackground(c);
b = new JButton("Click to choose your color");
b.addActionListener(
new ActionListener(){
public void actionPerformed(ActionEvent event){
c = JColorChooser.showDialog(null, "Pick your color", c);
if(c==null)
c=(Color.WHITE);
p.setBackground(c);
}
}
);
add(p, BorderLayout.CENTER);
add(b, BorderLayout.SOUTH);
setSize(450,200);
setVisible(true);
}
}
Let us move on and create our main class king.java
. This class will be used in executing our program.
Main class king.java
Like we already know, this is our main class which is responsible for the execution of our program.
The first thing to do on this new class is to import the swing
class which prepares the frame to be usable in our Java program.
import javax.swing.*;
That done, now we are going to create a new object to call our sub-class, tee.java
into the main class.
tee t = new tee();
That is it, we have successfully link our sub-class to our main class.
Now we are going to create the argument that will be responsible to terminating the program whenever we click on the close icon. To do that, we add the EXIT_ON_CLOSE
argument.
t.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
And that is the last line of code for our main class. Below is the full code for the king.java.
king.java Full Code
import javax.swing.*;
public class king {
public static void main(String[] args) {
tee t = new tee();
t.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
That is it guys! We have just created our program completely. Now let's run it and see how it looks.
Output
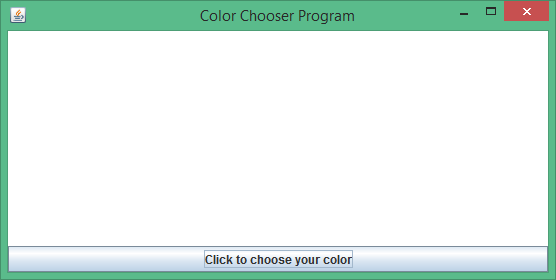
When the program is first run, the panel has our default background
WHITE
. The user can now click on the button to choose a color.
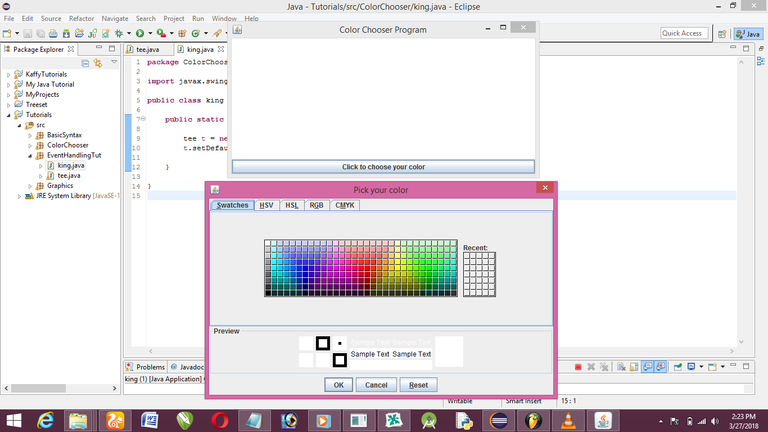
As you can see, as soon as the user click on the button, the JColorChooser comes up with variety of colors from which the user can pick from.
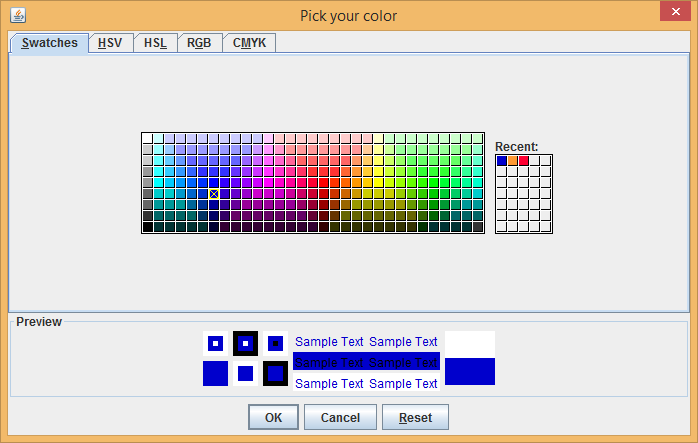
The preview dialog at the bottom of the window shows the color being picked by the user.
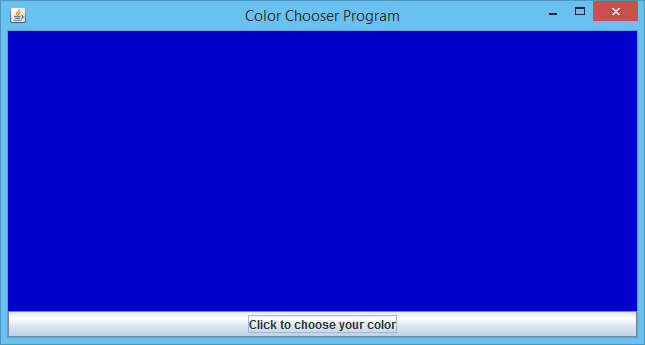
Once the user hit
Okay
, the color fill our JPnal which represents the window background.
Source Code On GitHut
The full source code is on my GitHub and it's available for you in case you need to try the program out.
Curriculum
- Java Graphical User Interface With JFrame: Drawing Graphics With Eclipse
- Java Graphical User Interface With JFrame: Event Handling And Action Listening
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Dear friend! Next time also use #wafrica and follow @wafrica to get an upvote on your quality posts!
Hey @teekingtv I am @utopian-io. I have just upvoted you!
Achievements
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x